- Understanding Cryptography Basics
- Cryptographic Hash Functions
- Properties of Hash Functions
- Password Hashing Techniques
- Salting
- Public and Private Keys
- Key Generation
- Exploring Blockchain Technology
- Blockchain Structure
- Block Components
- Implementing a Simple Blockchain
- Addressing Scalability Issues
- Increasing Block Size
- Off-Chain Transactions
- Strategies for Solving Specific Assignments
- Solving Password Hashing Problems
- Understanding Rainbow Tables
- Enhancing Password Security
- Cracking Passwords
- Brute-Force Attacks
- Dictionary Attacks
- Analyzing Blockchain Assignments
- Evaluating Transaction Verification
- Proof-of-Work and Scalability
- Proposing Scalability Solutions
- Sharding
- Layer 2 Solutions
- Conclusion
Cryptography and blockchain are two critical areas in the field of computer science, especially in the context of security and decentralized systems. Tackling assignments in these areas requires a strong understanding of both theoretical concepts and practical applications. This blog will help you approach such assignments methodically, providing insights into key concepts, practical examples, and strategies to solve common problems. Whether you're studying for exams or need network security assignment help, this guide offers invaluable resources to navigate through complex topics effectively.
Understanding Cryptography Basics
Cryptography is the foundation of secure communication in the digital world. It involves various techniques and methods to secure data and communications from unauthorized access. Understanding these basics is crucial for solving any cryptography-related assignment.
Cryptographic Hash Functions
A cryptographic hash function is a mathematical algorithm that transforms any input data into a fixed-size string of characters, which appears random. The output, often referred to as the 'digest,' is unique to each unique input, making it an essential tool in data integrity and security.
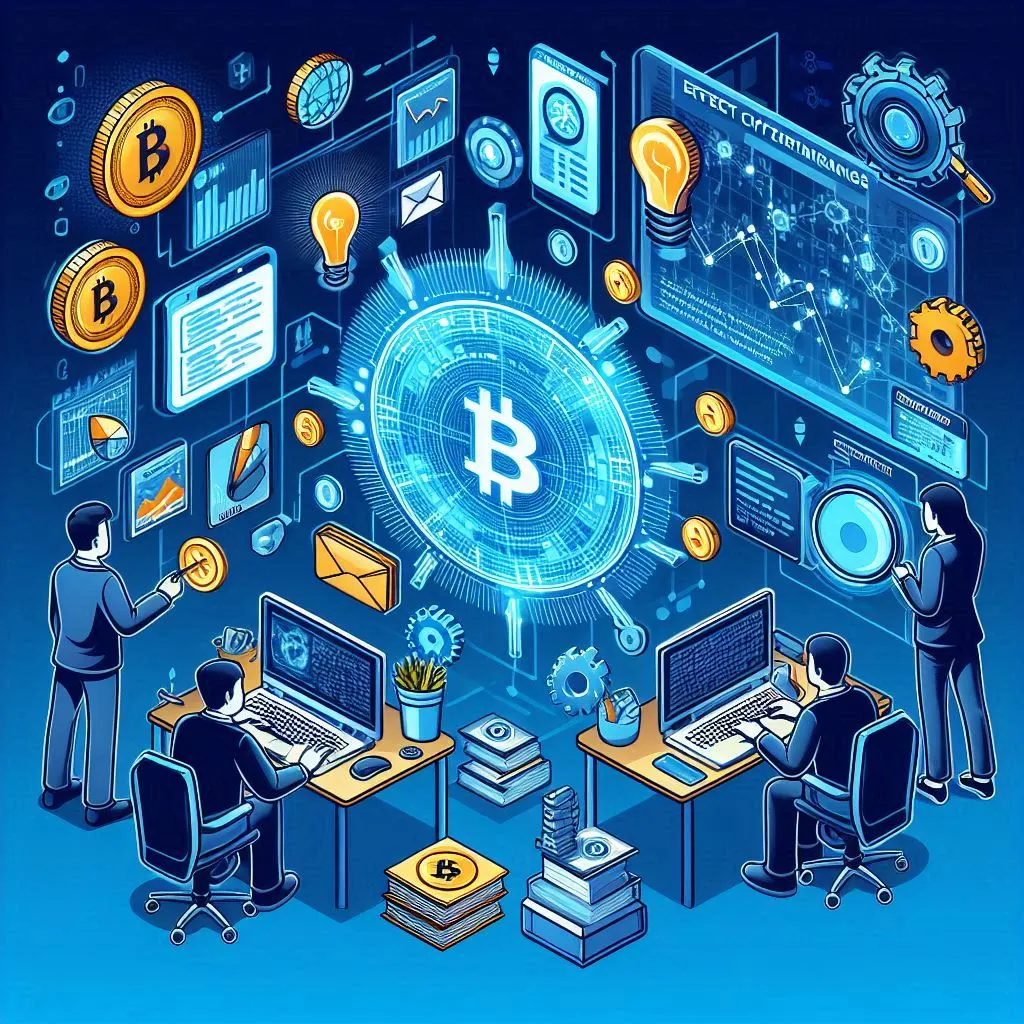
Properties of Hash Functions
- Deterministic:The same input always produces the same output.
- Fast Computation: Hashing a message is computationally efficient.
- Preimage Resistance: It should be infeasible to reverse-engineer the original input from its hash.
- Small Changes in Input Produce Large Changes in Output: A tiny alteration in input drastically changes the hash.
Password Hashing Techniques
Password hashing is a specific application of cryptographic hash functions. It is used to securely store passwords by converting them into a fixed-size string that cannot be easily reversed back to the original password.
Salting
Adding a random value, known as a salt, to the password before hashing ensures that identical passwords have different hashes, enhancing security against dictionary attacks and rainbow tables.
import os
import hashlib
def hash_password_with_salt(password):
salt = os.urandom(16)
pwdhash = hashlib.pbkdf2_hmac('sha256', password.encode(), salt, 100000)
return salt + pwdhash
password = "securepassword"
hashed_password = hash_password_with_salt(password)
print(hashed_password)
Public and Private Keys
Public key cryptography involves two keys: a public key, which can be shared openly, and a private key, which is kept secret. The public key encrypts data, while the private key decrypts it, ensuring secure communication and data transfer.
Key Generation
Key pairs are generated using cryptographic algorithms, ensuring that the private key cannot be derived from the public key. This asymmetry is the cornerstone of modern cryptographic systems.
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.primitives import serialization
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
)
public_key = private_key.public_key()
pem = private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption()
)
print(pem.decode('utf-8'))
Exploring Blockchain Technology
Blockchain technology underpins cryptocurrencies and other decentralized applications. It involves a chain of blocks, where each block contains a list of transactions. Understanding blockchain is essential for solving related assignments.
Blockchain Structure
A blockchain is a sequential chain of blocks, each containing a list of transactions. Each block has a unique hash and the hash of the previous block, creating a secure and immutable chain.
Block Components
1. Index: Position of the block within the chain.
- Previous Hash:Hash of the preceding block.
- Timestamp: Time of block creation.
- Data:Actual transaction data.
- Hash:Unique identifier for the block.
Implementing a Simple Blockchain
Implementing a basic blockchain can help solidify your understanding of how it works. Here's a simple Python implementation:
import hashlib
import time
class Block:
def __init__(self, index, previous_hash, timestamp, data, hash):
self.index = index
self.previous_hash = previous_hash
self.timestamp = timestamp
self.data = data
self.hash = hash
def calculate_hash(index, previous_hash, timestamp, data):
value = str(index) + previous_hash + str(timestamp) + data
return hashlib.sha256(value.encode()).hexdigest()
def create_genesis_block():
return Block(0, "0", int(time.time()), "Genesis Block", "0")
def create_new_block(previous_block, data):
index = previous_block.index + 1
timestamp = int(time.time())
hash = calculate_hash(index, previous_block.hash, timestamp, data)
return Block(index, previous_block.hash, timestamp, data, hash)
blockchain = [create_genesis_block()]
previous_block = blockchain[0]
num_blocks_to_add = 5
for i in range(0, num_blocks_to_add):
data = "Block #" + str(i + 1)
block_to_add = create_new_block(previous_block, data)
blockchain.append(block_to_add)
previous_block = block_to_add
for block in blockchain:
print(f"Block #{block.index} [Hash: {block.hash}]")
Addressing Scalability Issues
Blockchain scalability is a significant challenge, especially as the number of transactions grows. Several strategies can help address these issues.
Increasing Block Size
Larger blocks can store more transactions, reducing the need for frequent block creation and lowering transaction fees. However, this approach also increases the size of the blockchain, making it harder to store and transmit.
Off-Chain Transactions
Off-chain transactions involve transferring value outside of the blockchain, reducing the load on the main chain. Solutions like the Lightning Network use this approach to enable fast and low-cost transactions.
Strategies for Solving Specific Assignments
When faced with specific assignments in cryptography and blockchain, it's essential to break down the problems and apply the concepts you've learned. Here are strategies for some common types of assignments.
Solving Password Hashing Problems
Assignments involving password hashing often require implementing secure hashing techniques and understanding their vulnerabilities.
Understanding Rainbow Tables
Rainbow tables are precomputed tables used for reversing cryptographic hash functions, primarily to crack password hashes. Understanding their structure and limitations is crucial.
def create_rainbow_table(passwords):
table = {}
for password in passwords:
hash = hash_password(password)
table[hash] = password
return table
def crack_password(hash, rainbow_table):
return rainbow_table.get(hash, None)
passwords = ["password1", "password2", "password3"]
rainbow_table = create_rainbow_table(passwords)
cracked_password = crack_password("5e884898da28047151d0e56f8dc6292773603d0d6aabbdd2328ab60e45c4f5d", rainbow_table)
print(cracked_password)
Enhancing Password Security
To enhance password security, use techniques like salting and peppering. Salting involves adding random data to passwords before hashing, while peppering adds an additional secret value.
Cracking Passwords
Assignments that involve cracking passwords require a methodical approach and the use of appropriate tools and techniques.
Brute-Force Attacks
A brute-force attack tries every possible combination until it finds the correct one. While simple, it is often impractical for strong passwords due to the computational power required.
Dictionary Attacks
A dictionary attack uses a predefined list of potential passwords. This method is more efficient than brute-force but still limited by the comprehensiveness of the dictionary.
import hashlib
def crack_password(hash, dictionary):
for word in dictionary:
if hashlib.md5(word.encode()).hexdigest() == hash:
return word
return None
hashes = ["1f3870be274f6c49b3e31a0c6728957f", "fe01d67a002dfa0f3ac084298142eccd"]
dictionary = ["apple", "banana", "cherry"]
cracked_passwords = [crack_password(hash, dictionary) for hash in hashes]
print(cracked_passwords)
Analyzing Blockchain Assignments
Blockchain assignments often involve understanding the implications of certain design choices and proposing solutions to real-world problems.
Evaluating Transaction Verification
Assignments may require evaluating the efficiency of transaction verification processes and their impact on the overall system.
Proof-of-Work and Scalability
Proof-of-work (PoW) is the consensus mechanism used by Bitcoin to validate transactions and add new blocks to the blockchain. Understanding its implications on scalability and security is crucial.
Proposing Scalability Solutions
In assignments that require proposing solutions for blockchain scalability, consider different approaches and their trade-offs.
Sharding
Sharding involves splitting the blockchain into smaller, more manageable pieces, each capable of processing its own transactions. This method can significantly enhance scalability but requires complex coordination.
Layer 2 Solutions
Layer 2 solutions, such as the Lightning Network, operate on top of the main blockchain to handle transactions off-chain, reducing the load on the main chain.
Conclusion
Solving assignments in cryptography and blockchain requires a blend of theoretical knowledge and practical application. By understanding key concepts, implementing fundamental techniques, and applying strategic problem-solving methods, you can tackle these assignments with confidence. Remember, practice and continuous learning are key to mastering these complex subjects.
For personalized assistance and expert guidance, visit Computer Network Assignment Help. Our team of professionals is ready to help you with your assignments and deepen your understanding of cryptography and blockchain.